OAuth 2.0 Authorization Code Grant Flow
Public Apps
OAuth 2.0 code grant is a secure authorization framework that allows a user to grant a third-party application access to their resources without sharing their credentials. This is achieved by the application requesting authorization from the user, who then grants access by providing a code to the application. The application then exchanges this code for an access token, which it can use to access the user's resources on their behalf. This process helps to protect the user's sensitive information and ensures that only authorized applications can access their data.
In addition, this type of authentication is commonly used for public integrations designed for a wide audience. Any Event Temple customer can use a public integration, allowing for seamless and secure access to their resources while maintaining control over the applications that are authorized to access their data.
Flow
The authorization flow for OAuth2 includes the following steps:
- Redirect the user to the integration's authorization URL.
- Event Temple redirects the user to the integration's redirect_uri, which includes a code parameter.
- The integration exchanges the code for an access token and a refresh token
- The integration stores the refresh token and access token for future requests
This guide provides detailed instructions for each step of the OAuth2 flow, including relevant implementation details.
Step 1 - Redirect the user to the authorization URI
To initiate the authorization flow, redirect the user to the following URL:
<a href="https://client.eventtemple.com/oauth/authorize?
client_id=463558a3-725e-4f37-b6d3-0889894f68de&
redirect_uri=https%3A%2F%2Fexample.com%2Fauth%2Feventtemple%2Fcallback&
scope=crm_read,crm_manage&
response_type=code">Link to Event Temple</a>
The URL begins with https://client.eventtemple.com/oauth/authorize and includes the following parameters:
Parameter | Description |
---|---|
client_id | An identifier for your integration, found in the integration settings |
redirect_uri | The URL where the user should return after granting access. |
scope | Provides a way to limit the amount of access that is granted to an access token |
response_type | Oauth2 response type (should always be code) |
You can use the following provided assets to build your redirect URL link:

Redirect Button (long)
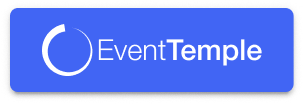
Redirect Button (compact)
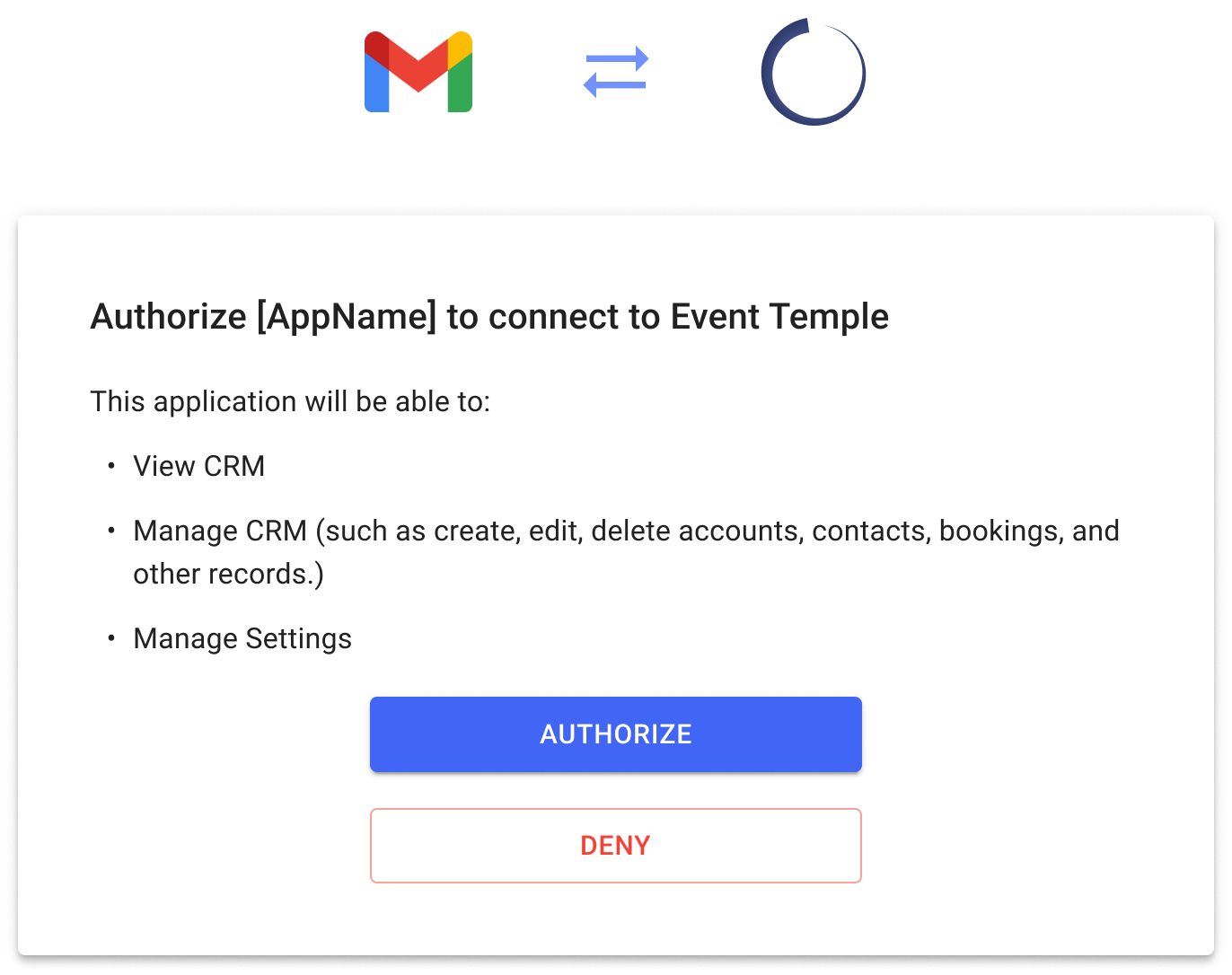
Authorization Page
Step 2 - Event Temple Redirects the user to the integration's redirect_uri, which includes a code parameter
After the user grants authorization, Event Temple redirects them to the provided redirect URI, which includes a code parameter. This code is a temporary authorization code that your integration will use to obtain an access token.
Step 3 - Exchange the code for an access token
To obtain an access token, your integration needs to exchange the temporary code for an access_token. Send a POST request to Event Temple's token URL: https://api.eventtemple.com/oauth/token.
The body of the request contains the following JSON-encoded fields:
client_id | An identifier for your integration, found in the integration settings |
client_secret | A secret for your integration, found in the integration settings |
code | The temporary authorization code obtained from the redirect URI |
redirect_uri | The same redirect URI used in Step 2 |
grant_type | The string "authorization_code" |
POST /oauth/token HTTP/1.1
Content-Type: application/json
{
"grant_type":"authorization_code",
"code":"e202e8c9-0990-40af-855f-ff8f872b1ec6",
"redirect_uri":"https://example.com/auth/notion/callback",
"client_id": "HZfCimov_5qG6XU2ZeqToBTGsnqmiM-UPaHMYhUDedg",
"client_secret": "xt2PAwpKQo0KVNXyBz4uvEcPYkT1oGFDE_1mUd-lHDY"
}
Event Temple will respond with an access_token, refresh_token, and additional information. Your integration should store the access_token securely and use it in future requests to access Event Temple's API on behalf of the authorized user.
Parameter | Description |
---|---|
access_token | A token that your integration uses to authenticate with Event Temple API. |
refresh_token | A token that your integration uses to obtain a new access_token when the previous one has expired. |
{
"access_token": "DRXLNWNguoWQNt2gdUwQTWDWwm4Tu0ay6cANCG1ABXY",
"token_type": "Bearer",
"expires_in": 7200,
"refresh_token": "e3sJawKmOR_rU5LhVDDCVFKlt_Mli9Vt52Sf9awzHt0",
"scope": "crm_manage crm_read",
"created_at": 1677539644
}
Step 4: The integration stores the access_token and refresh_token for future requests
You need to set up a way for your integration to store all of the access_token's that it receives, so that the integration can then choose a bearer token from storage based on the user it’s acting on behalf of for each request to the Notion API.
Making Requests with Access Token
Once you have obtained an access token, you can use it to make authenticated requests to the Event Temple API on behalf of a user.
To make an authenticated request, you'll need to include the access token in the Authorization header of the request using the Bearer authentication scheme. Here's an example request using the curl command-line tool:
GET https://api.eventtemple.com/v2/bookings HTTP/1.1
Authorization: Bearer <access_token>
X-API-ORG: <api_org_id>
Refreshing the Access Token
Refreshing a token is a critical part of OAuth 2.0 authentication flow, as it allows your integration to obtain a new access token without requiring the user to re-authenticate. When a user authenticates your integration for the first time, the authorization server issues an access token and a refresh token. The access token is short-lived and will expire after a certain period of time, whereas the refresh token is long-lived and can be used to obtain a new access token when the old one expires.
To refresh a token, your integration should make a POST request to the /oauth/token
endpoint of the authorization server with the following parameters:
Parameter | Description |
---|---|
client_id | The client ID of your integration. |
client_secret | The client secret of your integration. |
refresh_token | The refresh token that was obtained during the initial authentication flow. |
grant_type | The OAuth grant type, which should be set to "refresh_token". |
POST https:://api.eventtmeple.com/oauth/token HTTP/1.1
Content-Type: application/json
{
"grant_type": "refresh_token",
"refresh_token": "jvFwZ458HdMJXGjtRyGTskfdIv5bUGhIfEejGJJ2xW4",
"client_id": "HZfCimov_5qG6XU2ZeqToBTGsnqmiM-UPaHMYhUDedg",
"client_secret": "xt2PAwpKQo0KVNXyBz4uvEcPYkT1oGFDE_1mUd-lHDY"
}
Revoking a Token
Revoking a token is a way to invalidate an access or refresh token before its expiration time. This can be useful if a user revokes access to your application or if a token has been compromised. To revoke a token, send a POST request to the /oauth/revoke
endpoint with the following parameters:
Parameter | Description |
---|---|
client_id | The client ID of your integration. |
client_secret | The client secret of your integration. |
token | The access or refresh token to revoke. |
POST https:://api.eventtmeple.com/oauth/revoke HTTP/1.1
Content-Type: application/json
{
"token": "DRXLNWNguoWQNt2gdUwQTWDWwm4Tu0ay6cANCG1ABXY",
"client_id": "HZfCimov_5qG6XU2ZeqToBTGsnqmiM-UPaHMYhUDedg",
"client_secret": "xt2PAwpKQo0KVNXyBz4uvEcPYkT1oGFDE_1mUd-lHDY"
}
Updated about 2 months ago